Swift Development
Android app development involves creating applications for devices running on the Android operating system, one of the world’s most popular platforms. Developed by Google, Android powers various devices, from smartphones to wearables. Its open-source nature allows developers to create diverse apps catering to various user needs, making it a preferred choice for businesses and individuals.
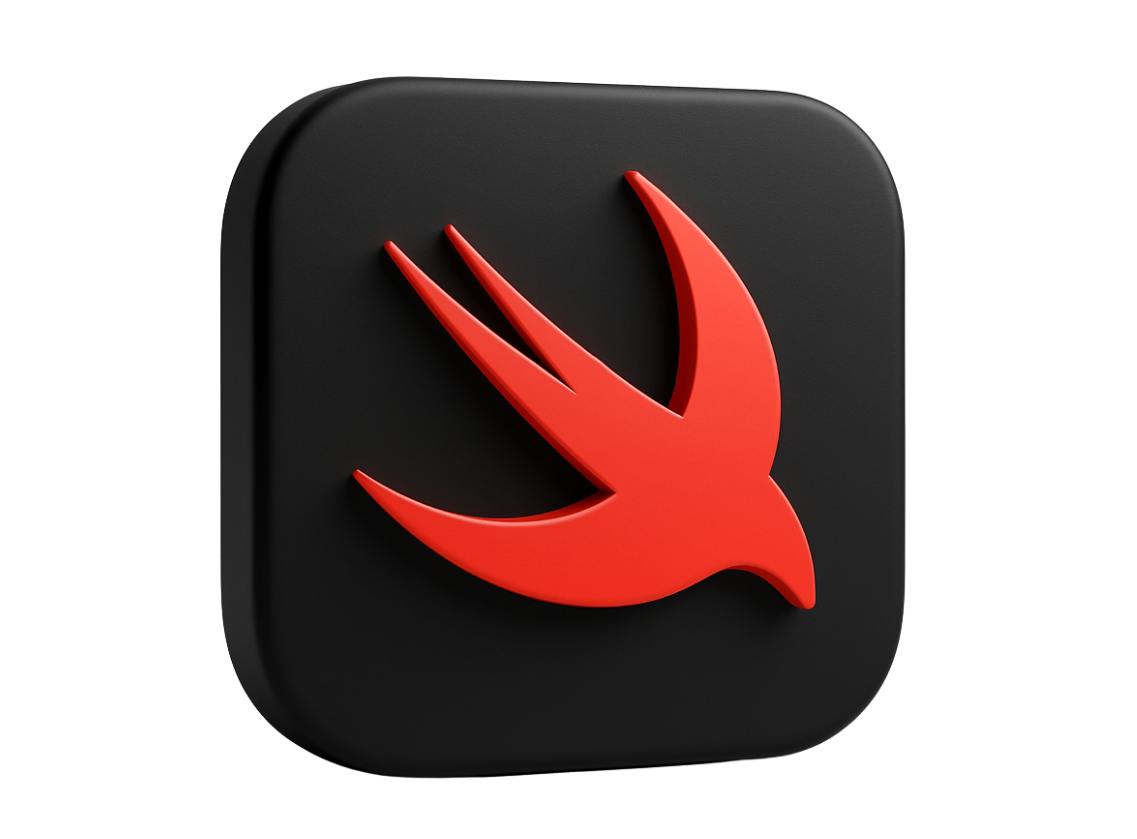